1. vector 컨테이너
표준 시퀀스 컨테이너 : 원소가 자신만의 insert 위치(순서)를 가지는 컨테이너 (선형) ( vector, deque, list )
배열 기반 컨테이너 : 데이터 여러 개가 하나의 메모리 단위에 저장 ( vector, deque )
노드 기반 컨테이너 : 각각의 데이터를 메모리 단위에 저장 ( list, set, multiset, map, multimap )
표준 연관 컨테이너 : 저장되는 원소가 삽입 순서와 달라도 특정 정렬 기준에 의해 자동 정렬(비선형) ( set, multiset, map, multimap )

2. 대표 함수
- push_back : 벡터 끝에 요소를 추가
- insert : 요소 또는 요소의 수를 벡터의 지정된 위치에 삽입
- assign : 벡터를 지우고 지정된 요소를 빈 벡터에 복사
- reserve : 벡터 개체에 대한 스토리지의 최소 길이를 예약
- resize : 벡터의 새 크기를 지정
- swap : 두 벡터의 요소를 교환
- emplace : 내부에서 생성된 요소를 벡터의 지정된 위치에 삽입
- emplace_back : 내부에서 생성된 요소를 벡터의 끝에 추가
3. 함수 예제
1. push_back
#include <iostream>
#include <vector>
using namespace std;
void main ()
{
vector<int> vtTest01;
vtTest01.push_back( 1 ); // 원소 입력
vtTest01.push_back( 2 );
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
system( "pause" );
}
결과
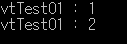
2. insert
#include <iostream>
#include <vector>
using namespace std;
void main ()
{
vector<int> vtTest01;
// ...
vector<int>::iterator itr;
itr = vtTest01.begin();
itr++;
vtTest01.insert( itr, 3 ); // itr가 가리키는 위치에 원소 3 입력
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
itr = vtTest01.begin();
itr++;
vtTest01.insert( itr, 3, 4 ); // itr가 가리키는 위치에 3개의 원소 4 입력
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
system( "pause" );
}
결과
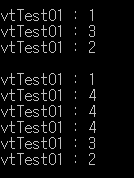
3. assign
#include <iostream>
#include <vector>
using namespace std;
void main ()
{
vector<int> vtTest01;
// ...
vector<int>::iterator itr;
// ...
vtTest01.assign( 6, 5 ); //6개의 원소 5을 할당
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
system( "pause" );
}
결과
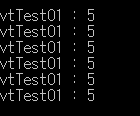
4. reserve
#include <iostream>
#include <vector>
using namespace std;
void main ()
{
vector<int> vtTest01;
// ...
vector<int>::iterator itr;
// ...
// ...
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
vtTest01.reserve( 10 ); // vector 예약 할당
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
for ( int iVal : vtTest01 )
cout << "iVal : " << iVal << endl;
cout << endl;
system( "pause" );
}
결과
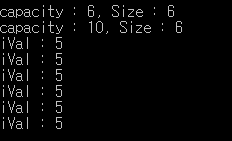
5. resize
#include <iostream>
#include <vector>
using namespace std;
void main ()
{
vector<int> vtTest01;
// ...
vector<int>::iterator itr;
// ...
// ...
// ...
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
vtTest01.resize( 4 ); // vector size 변경
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
for ( int iVal : vtTest01 )
cout << "iVal : " << iVal << endl;
cout << endl;
system( "pause" );
}
결과
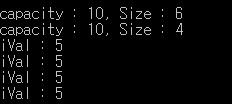
6. swap
#include <iostream>
#include <vector>
using namespace std;
void main ()
{
vector<int> vtTest01;
// ...
vector<int>::iterator itr;
// ...
// ...
// ...
// swap 테스트
vector<int> vtTest02;
for ( int iLoop = 0 ; iLoop < 10 ; iLoop++ )
vtTest02.push_back( iLoop );
for ( int iVal : vtTest02 )
cout << "vtTest02 : " << iVal << endl;
cout << endl;
vtTest02.swap( vtTest01 );
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
for ( int iVal : vtTest02 )
cout << "vtTest02 : " << iVal << endl;
cout << endl;
system( "pause" );
}
결과
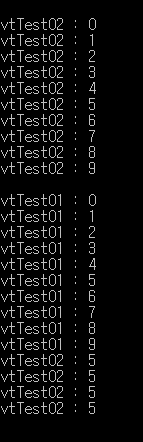
7. emplace
#include <iostream>
#include <vector>
using namespace std;
class CTest
{
private:
int m_iIndex;
public :
CTest( int iIndex ) : m_iIndex(iIndex)
{
cout << "생성자 호출(" << m_iIndex << ")" << endl;
}
CTest( CTest &clsItem ) : m_iIndex( clsItem.m_iIndex )
{
cout << "복사 생성자 호출(" << m_iIndex << ")" << endl;
}
CTest( CTest &&clsItem ) : m_iIndex( clsItem.m_iIndex )
{
cout << "이동 생성자 호출(" << m_iIndex << ")" << endl;
}
~CTest()
{
cout << "소멸자 호출(" << m_iIndex << ")" << endl;
}
};
void main ()
{
vector<int> vtTest01;
// ...
vector<int>::iterator itr;
// ...
// ...
// ...
// swap 테스트
vector<int> vtTest02;
// ...
// emplace
{
vector < CTest > vtTest03;
vtTest03.push_back( 0 );
//vtTest03.emplace_back( 1 );
cout << endl;
}
system( "pause" );
}
결과
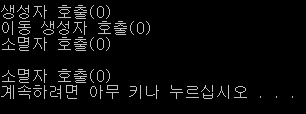
push_back일 경우
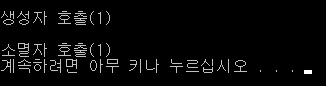
emplace_back일 경우
4. 전체 소스
#include <iostream>
#include <vector>
using namespace std;
class CTest
{
private:
int m_iIndex;
public :
CTest( int iIndex ) : m_iIndex(iIndex)
{
cout << "생성자 호출(" << m_iIndex << ")" << endl;
}
CTest( CTest &clsItem ) : m_iIndex( clsItem.m_iIndex )
{
cout << "복사 생성자 호출(" << m_iIndex << ")" << endl;
}
CTest( CTest &&clsItem ) : m_iIndex( clsItem.m_iIndex )
{
cout << "이동 생성자 호출(" << m_iIndex << ")" << endl;
}
~CTest()
{
cout << "소멸자 호출(" << m_iIndex << ")" << endl;
}
};
void main ()
{
vector<int> vtTest01;
vtTest01.push_back( 1 ); // 원소 입력
vtTest01.push_back( 2 );
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
vector<int>::iterator itr;
itr = vtTest01.begin();
itr++;
vtTest01.insert( itr, 3 ); // itr가 가리키는 위치에 원소 3 입력
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
itr = vtTest01.begin();
itr++;
vtTest01.insert( itr, 3, 4 ); // itr가 가리키는 위치에 3개의 원소 4 입력
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
vtTest01.assign( 6, 5 ); //6개의 원소 5을 할당
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
cout << endl;
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
vtTest01.reserve( 10 ); // vector 예약 할당
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
for ( int iVal : vtTest01 )
cout << "iVal : " << iVal << endl;
cout << endl;
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
vtTest01.resize( 4 ); // vector size 변경
cout << "capacity : " << vtTest01.capacity() << ", Size : " << vtTest01.size() << endl;
for ( int iVal : vtTest01 )
cout << "iVal : " << iVal << endl;
cout << endl;
// swap 테스트
vector<int> vtTest02;
for ( int iLoop = 0 ; iLoop < 10 ; iLoop++ )
vtTest02.push_back( iLoop );
for ( int iVal : vtTest02 )
cout << "vtTest02 : " << iVal << endl;
cout << endl;
vtTest02.swap( vtTest01 );
for ( int iVal : vtTest01 )
cout << "vtTest01 : " << iVal << endl;
for ( int iVal : vtTest02 )
cout << "vtTest02 : " << iVal << endl;
cout << endl;
// emplace
{
vector < CTest > vtTest03;
//vtTest03.push_back( 0 );
vtTest03.emplace_back( 1 );
cout << endl;
}
system( "pause" );
}