OpenCV에서 Point_와 Size_ 클래스에 대해 알아보겠습니다.
Point_
우선 Point_클래스 부터 알아보겠습니다.
Point_는 2차원 평면의 점의 좌표를 표현하는 클래스입니다. 해당 클래스는 템플릿 클래스이기 때문에 사용할 때 자료형을 명시해야합니다. 예를 들면 정수(int)형일 경우 Point_<int>로 명시하여 사용합니다.
OpenCV에서는 아래와 같이 tyedef도 제공합니다.
template<typename _Tp> class Point_
{
public:
typedef _Tp value_type;
//! default constructor
Point_();
Point_(_Tp _x, _Tp _y);
Point_(const Point_& pt);
Point_(Point_&& pt) CV_NOEXCEPT;
Point_(const Size_<_Tp>& sz);
Point_(const Vec<_Tp, 2>& v);
Point_& operator = (const Point_& pt);
Point_& operator = (Point_&& pt) CV_NOEXCEPT;
//! conversion to another data type
template<typename _Tp2> operator Point_<_Tp2>() const;
//! conversion to the old-style C structures
operator Vec<_Tp, 2>() const;
//! dot product
_Tp dot(const Point_& pt) const;
//! dot product computed in double-precision arithmetics
double ddot(const Point_& pt) const;
//! cross-product
double cross(const Point_& pt) const;
//! checks whether the point is inside the specified rectangle
bool inside(const Rect_<_Tp>& r) const;
_Tp x; //!< x coordinate of the point
_Tp y; //!< y coordinate of the point
};
typedef Point_<int> Point2i;
typedef Point_<int64> Point2l;
typedef Point_<float> Point2f;
typedef Point_<double> Point2d;
typedef Point2i Point;
Point 클래스를 사용한 예제는 아래와 같습니다.
#include <iostream>
#include "opencv2/opencv.hpp"
#ifdef _DEBUG
#pragma comment(lib, "opencv_world440d.lib")
#else
#pragma comment(lib, "opencv_world440.lib")
#endif
using namespace std;
using namespace cv;
int main(void)
{
Point p1;
p1.x = 1; p1.y = 5; // x, y에 직접 대입
Point p2(10, 2); // 생성자에 좌표 대입
Point p3 = p1 + p2; // 더하기 연산 가능
Point p4 = p3 * 2; // *연산 가능
int d1 = p1.dot(p2); // p1, p2 내적 계산
bool b1 = (p1 == p2);
cout << "p1: " << p1 << endl;
cout << "p2: " << p2 << endl;
cout << "p3: " << p3 << endl;
cout << "p4: " << p4 << endl;
cout << "d1: " << d1 << endl;
cout << "b1: " << b1 << endl;
system("pause");
return 0;
}
출력 결과는 아래와 같습니다.
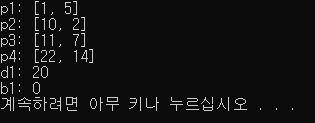
Size_
다음으로 Size_ 클래스 입니다.
Size_는 영상에서, 혹은 사각형에서 영역의 크기를 표현할 때 사용합니다.
Point_와 마찬가지로 템플릿 클래스이며 사용할 때 자료형을 명시해야합니다.
template<typename _Tp> class Size_
{
public:
typedef _Tp value_type;
//! default constructor
Size_();
Size_(_Tp _width, _Tp _height);
Size_(const Size_& sz);
Size_(Size_&& sz) CV_NOEXCEPT;
Size_(const Point_<_Tp>& pt);
Size_& operator = (const Size_& sz);
Size_& operator = (Size_&& sz) CV_NOEXCEPT;
//! the area (width*height)
_Tp area() const;
//! aspect ratio (width/height)
double aspectRatio() const;
//! true if empty
bool empty() const;
//! conversion of another data type.
template<typename _Tp2> operator Size_<_Tp2>() const;
_Tp width; //!< the width
_Tp height; //!< the height
};
typedef Size_<int> Size2i;
typedef Size_<int64> Size2l;
typedef Size_<float> Size2f;
typedef Size_<double> Size2d;
typedef Size2i Size;
Size_ 클래스의 실제 사용 예시는 아래와 같습니다.
#include <iostream>
#include "opencv2/opencv.hpp"
#ifdef _DEBUG
#pragma comment(lib, "opencv_world440d.lib")
#else
#pragma comment(lib, "opencv_world440.lib")
#endif
using namespace std;
using namespace cv;
// Size: 사각형 영역 표시
int main(void)
{
Size sz1;
Size sz2(100, 200); // 100 x 200
Size sz3 = sz1 + sz2;
Size sz4 = sz3 * 2; // 200 x 400
int area = sz4.area(); // 면적: 80000
cout << "sz1: " << sz1 << endl;
cout << "sz2: " << sz2 << endl;
cout << "sz3: " << sz3 << endl;
cout << "sz4: " << sz4 << endl;
cout << "area: " << area << endl;
system("pause");
return 0;
}
Size_ 클래스는 면적을 계산하는 area 함수를 제공합니다.
출력 결과는 아래와 같습니다.
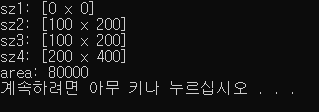