이번 글에서는 QMap에 대해 알아보겠습니다.
QMap 클래스도 자료구조에서 맵을 구현한 클래스입니다.
맵은 키(Key)와 값(Value)이 쌍을 이루며 하나의 키에는 하나의 값이 매핑이 됩니다.
맵은 키를 통해 값에 접근할 수 있게 하는 자료구조인데 그렇기 때문에 키는 중복을 허용하지 않습니다.(값은 중복 가능합니다.)
더 자세한 개념은 구글에 검색해보시길 바랍니다.
QMap 선언
QMap 선언 방법은 아래와 같습니다.
QMap<Key, T>
Key(키)와 T(값)에 자료형을 입력하여 선언합니다.
// QMap 선언
QMap mapIntString;
키, 값 삽입
키, 값을 삽입하는 방법은 insert 함수를 사용하거나 operator[] 함수를 사용하시면 됩니다.
// 키, 값 삽입
mapIntString.insert(1, "one");
mapIntString.insert(2, "two");
mapIntString[3] = "three";
mapIntString[5] = "five";
값 얻기
삽입한 키, 값을 반대로 얻는 방법에 대해 알아보겠습니다.
키로 값을 얻는 방법은 value 함수를 사용하거나 operator[] 함수를 사용하면 됩니다.
// 키로 값 얻기
QString strValue1 = mapIntString[1];
QString strValue2 = mapIntString.value(2);
값 얻기 - 기본값
없는 키로 값을 얻으면 어떻게 될까요?
QString strValue4 = mapIntString.value(4); // 키 4는 없음
이 경우 값에는 아무런 값도 들어 있지 않습니다.
value 함수 두 번째 인자에 키가 없을 경우를 대비해 값을 넣어두면 없는 키의 기본값을 얻을 수 있습니다.
// 키로 값 얻기 - 키가 없으면 기본값 반환
QString strValue3= mapIntString.value(3, "셋"); // 없으면 "셋" 반환. Key 3이 있으므로 three 반환
QString strValue4 = mapIntString.value(4, "넷"); // Key 4가 없으므로 "넷" 반환
키 존재 유무 확인
키 존재 유무는 contains 함수를 사용하여 알 수 있습니다.
// 키 존재 유무 확인
if (mapIntString.contains(4))
qDebug() << "Key 4 is Exist. Value: " << mapIntString.value(4);
else
qDebug() << "Key 4 is Not Exist.";
반복문
맵 클래스에 입력한 모든 내용을 반복문으로도 확인 할 수 있습니다.
첫번째 방법은 QMapIterator를 사용하는 방법입니다.
// 반복문 1
QMapIteratoritr(mapIntString);
while (itr.hasNext())
{
itr.next();
qDebug() << "Key: " << itr.key() << ", Value: " << itr.value();
}
두번째로 const_iterator를 이용하는 방법도 있습니다.
// 반복문 2
for (QMap<int, QString>::const_iterator itr = mapIntString.cbegin(), end = mapIntString.cend(); itr != end; ++itr)
qDebug() << "Key: " << itr.key() << ", Value: " << itr.value();
키 삭제
키를 삭제하는 방법은 remove 함수를 사용하시면 됩니다.
// 키 삭제
mapIntString.remove(1);
mapIntString.remove(3);
mapIntString.remove(4); // 4는 없는 Key
전체 코드는 아래와 같습니다.
#include <QCoreApplication>
#include <QMap>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// QMap 선언
QMap<int, QString> mapIntString;
// 키, 값 삽입
qDebug() << "=========== 키, 값 삽입";
mapIntString.insert(1, "one");
mapIntString.insert(2, "two");
mapIntString[3] = "three";
mapIntString[5] = "five";
qDebug() << mapIntString;
// 키로 값 얻기
qDebug() << "=========== 키로 값 얻기";
QString strValue1 = mapIntString[1];
QString strValue2 = mapIntString.value(2);
qDebug() << "strValue1: " << strValue1;
qDebug() << "strValue2: " << strValue2;
// 키로 값 얻기 - 키가 없으면 기본값 반환
qDebug() << "키로 값 얻기 - 키가 없으면 기본값 반환";
QString strValue3= mapIntString.value(3, "셋"); // 없으면 "셋" 반환. Key 3이 있으므로 three 반환
QString strValue4 = mapIntString.value(4, "넷"); // Key 4가 없으므로 "넷" 반환
qDebug() << "strValue3: " << strValue3;
qDebug() << "strValue4: " << strValue4;
// 키 존재 유무 확인
qDebug() << "=========== 키 존재 유무 확인";
if (mapIntString.contains(4))
qDebug() << "Key 4 is Exist. Value: " << mapIntString.value(4);
else
qDebug() << "Key 4 is Not Exist.";
// 반복문 1
qDebug() << "=========== 반복문 1";
QMapIterator<int, QString>itr(mapIntString);
while (itr.hasNext())
{
itr.next();
qDebug() << "Key: " << itr.key() << ", Value: " << itr.value();
}
// 반복문 2
qDebug() << "=========== 반복문 2";
for (QMap<int, QString>::const_iterator itr = mapIntString.cbegin(), end = mapIntString.cend(); itr != end; ++itr)
qDebug() << "Key: " << itr.key() << ", Value: " << itr.value();
// 키 삭제
qDebug() << "=========== 키 삭제";
mapIntString.remove(1);
mapIntString.remove(3);
mapIntString.remove(4); // 4는 없는 Key
qDebug() << mapIntString;
qDebug() << "===========";
return a.exec();
}
실행 결과입니다.
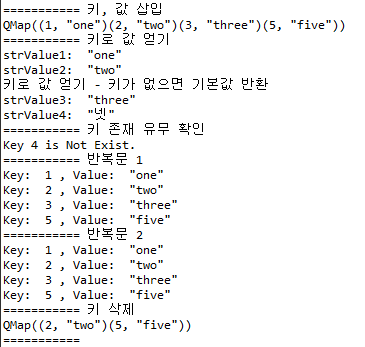
이상으로 QMap에 대해 알아보았습니다.
- 참고문서
- https://doc.qt.io/qt-6/qmap.html