이번 글에서는 클래스 템플릿에 대해 알아보겠습니다.
클래스 템플릿도 함수 템플릿처럼 만드시면 됩니다.
// class template
template <typename T>
class A
{
private:
T a;
public:
A( T a ) : a( a ) {}
void SetA( T a ) { this->a = a; }
T GetA() { return this->a; }
};
클래스 멤버 변수나 함수에 템플릿을 지정해주셔서 위와 같이 사용하시면 됩니다.
만약 클래스 내부에 멤버 함수를 선언하고, 외부에 멤버 함수를 정의하면 아래와 같이 코딩해야 합니다.
// class template
template <typename T>
class A
{
private:
T a;
public:
A( T a ) : a( a ) {}
void SetA( T a ) { this->a = a; }
T GetA() { return this->a; }
void Print();
};
// 클래스 외부에 함수 정의
template <typename T>
void A<T>::Print() { cout << this->a << endl; }
템플릿 매개변수를 여러 개 지정할 수 있습니다. 템플릿 매개변수 T 이외에 다른 템플릿 매개변수를 추가하고 싶으시면 아래와 같이 작업합니다.
// class template
template <typename T1, typename T2>
class A
{
private:
T1 a;
T2 b;
public:
A( T1 a, T2 b ) : a( a ), b( b ) {}
void SetA( T1 a ) { this->a = a; }
T1 GetA() { return this->a; }
void SetB( T2 b ) { this->b = b; }
T2 GetB() { return this->b; }
void Print();
};
// 클래스 외부에 함수 정의
template <typename T1, typename T2>
void A<T1, T2>::Print() { cout << this->a << endl << this->b << endl; }
전체 코드는 아래와 같습니다.
#include <iostream>
#include <string>
using namespace std;
// 함수 템플릿
// class, typename 둘다 사용 가능
template <typename T>
T Sum( T A, T B )
{
T C = A + B;
cout << "Sum : " << C << endl;
return C;
}
// 인자가 2개
template <typename T1, typename T2>
void printAll( T1 A, T2 B )
{
cout << "T1 : " << A << endl;
cout << "T2 : " << B << endl;
}
// template 특수화
template <>
char* Sum<char*> ( char* s1, char* s2 )
{
char* str = "[char*] template";
cout << s1 << " " << s2 << endl;
return str;
}
// class template
template <typename T1, typename T2>
class Person
{
private:
string name;
T1 height;
T2 age;
public:
Person( string name, T1 height, T2 age ) : name( name ), height( height ), age( age ) {}
void SetName( string name ) { this->name = name; }
void SetHeight( T1 height ) { this->height = height; }
void SetAge( T2 age ) { this->age = age; }
void PrintInfo1() { cout << "1 name : " << this->name << ", height : " << this->height << ", age : " << this->age << endl; }
void PrintInfo2();
};
// 외부 선언
template <typename T1, typename T2>
void Person<T1, T2>::PrintInfo2() { cout << "2 name : " << this->name << ", height : " << this->height << ", age : " << this->age << endl; }
int main()
{
// 일반적임 함수 템플릿
float fA = 4.3f, fB = 6.2f;
Sum<float>( fA, fB );
short sA = 4, sB = 1;
Sum<short>( sA, sB );
cout << endl;
// 서로 다른 데이터 형으로 인자가 2개
string str1 = "hellow C++ ";
int iB = 30;
printAll<string, int>( str1, iB );
cout << endl;
// template 특수화 : 재정의 하고싶을때
char *s1 = "hellow C++";
char *s2 = "world";
Sum<char *>( s1, s2 );
cout << endl;
// class template
Person<int, string> p1( "David", 180, "10살" );
Person<string, int> p2( "Lachel", "165cm", 20 );
p1.PrintInfo1();
p2.PrintInfo1();
cout << endl;
// class 멤버 함수 class 외부 정의
p1.PrintInfo2();
p2.PrintInfo2();
system( "pause" );
return 0;
}
실행 결과
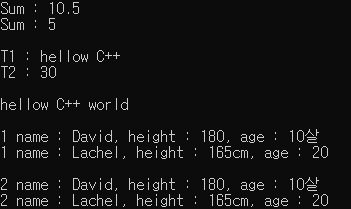