마지막으로 Mat 클래스의 연산과 변환에 대해 알아보겠습니다.
OpenCV에서는 Mat 클래스의 표현과 연산을 위한 기능도 제공하고있습니다.
mat.hpp에 보면 아래 그림과 같이 +, -, *, / 뿐만 아니라 비교, 논리 연산도 제공합니다.
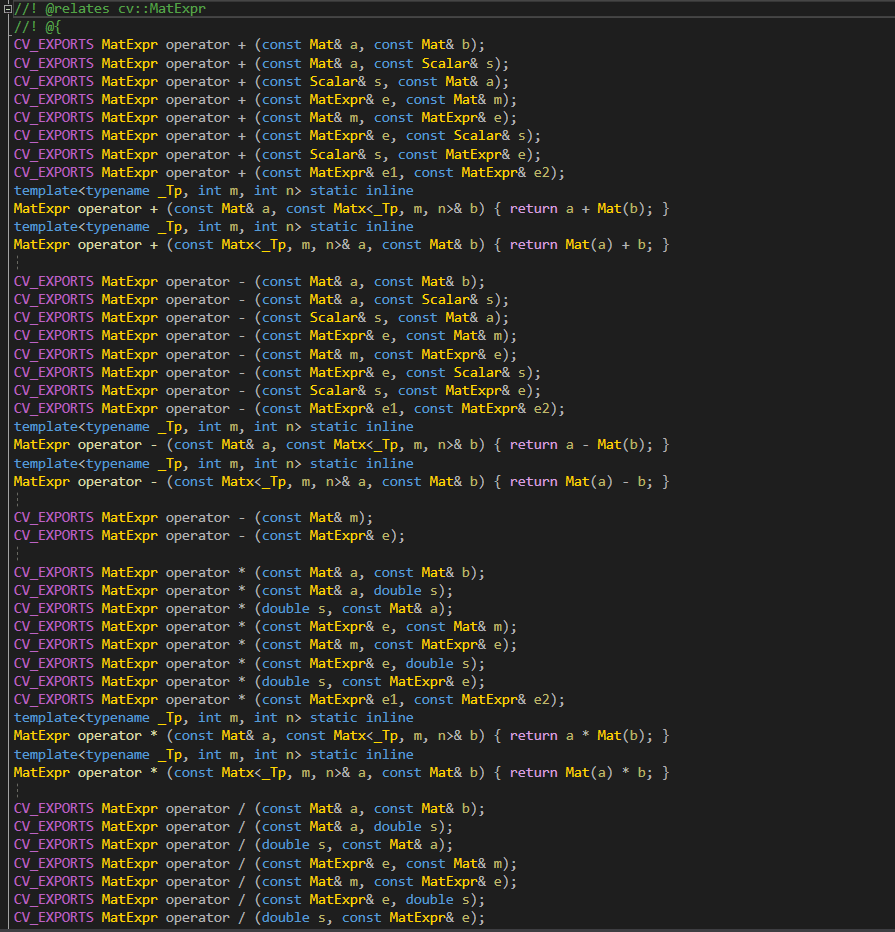
MatExpr은 OpenCV의 Mat 클래스의 연산을 할 때 사용되는 클래스입니다.
Mat뿐만 아니라 Scalar 클래스도 연산에 가능하며 int, float같은 자료형도 사용 가능합니다.
행렬의 곱은 아래 mul 함수로 사용 가능합니다.
MatExpr mul(InputArray m, double scale=1) const;
역행렬을 구할 땐 아래 inv함수를 사용합니다.
MatExpr inv(int method=DECOMP_LU) const;
행렬의 행과 열을 교환하는 전치 행렬은 t함수를 사용합니다.(MxN 함수를 NxM으로 변경)
MatExpr t() const;
위 함수들의 예제 코드는 아래와 같습니다.
#include <iostream>
#include "opencv2/opencv.hpp"
using namespace std;
using namespace cv;
int main()
{
float afMat[] = { 1,2,3,4 };
Mat mat_1( 2, 2, CV_32FC1, afMat );
cout << "mat_1 : \n" << mat_1 << endl;
Mat mat_2 = mat_1.inv();
cout << "mat_2 : \n" << mat_2 << endl;
cout << "mat_1.t() : \n" << mat_1.t() << endl;
cout << "mat_2 + 5 : \n" << mat_2 + 5 << endl;
return 0;
}
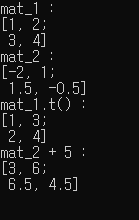
행렬의 타입을 변환하기 위해서는 아래 convertTo 함수를 사용합니다.
void convertTo( OutputArray m, int rtype, double alpha=1, double beta=0 ) const;
convertTo 함수는 CV_8UC1을 CV_32FC1으로 변경 가능 하고 uchar 형을 float, double 형으로도 변환 가능합니다.
행렬의 크기 또는 채널수를 변경할 때 reshape함수를 사용합니다.
Mat reshape(int cn, int rows=0) const;
Mat reshape(int cn, int newndims, const int* newsz) const;
Mat reshape(int cn, const std::vector<int>& newshape) const;