이번 글에서는 클래스 상속을 이용한 간단한 WinForm 예제 글 입니다.
아래와 같이 WinForm을 생성한 뒤 ListBox, Button, TextBox를 생성해 줍니다.
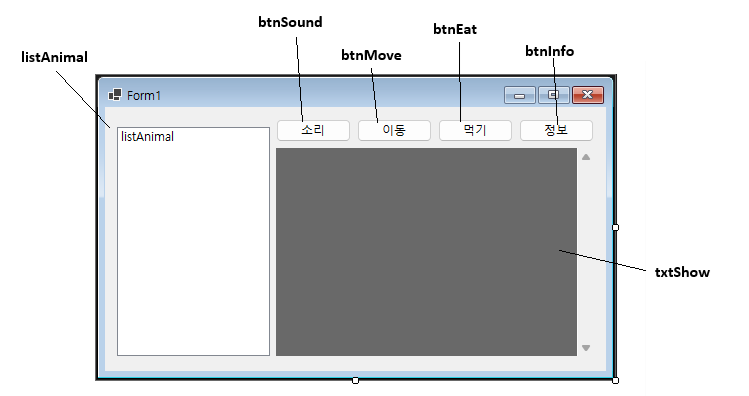
그 다음 Form을 더블 클릭 하여 Load 이벤트 함수를 만들어 줍니다. Form1_Load함수에는 리스트박스 초기화에 대한 코드를 적어줍니다.
// Form1.cs
namespace class_basic
{
public partial class Form1 : Form
{
private void Form1_Load(object sender, EventArgs e)
{
listAnimal.Items.Clear();
listAnimal.Items.Add(new AnimalListViewItem(new CPerson()));
listAnimal.Items.Add(new AnimalListViewItem(new CDog()));
listAnimal.Items.Add(new AnimalListViewItem(new CBird()));
}
}
}
리스트 박스 선택에 대한 SelectedValueChanged 이벤트 함수도 만들어 줍니다. 리스트 박스 선택 후 SelectedValueChanged를 더블 클릭 하면 아래와 같이 코드가 생성됩니다.
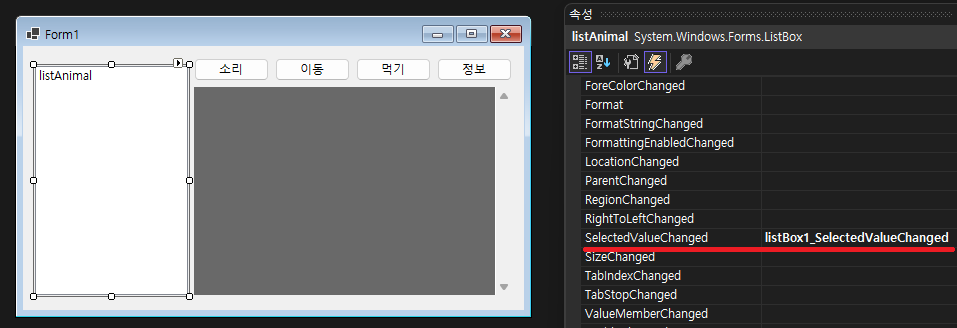
// Form1.cs
namespace class_basic
{
public partial class Form1 : Form
{
private void listBox1_SelectedValueChanged(object sender, EventArgs e)
{
if (sender.Equals(listAnimal) && listAnimal.SelectedItem != null)
{
AnimalListViewItem item = (AnimalListViewItem)listAnimal.SelectedItem;
CAnimal anim = item.Animal;
selectedAnimal = anim;
string str = "선택: " + anim.Name + Environment.NewLine;
txtShow.AppendText(str);
}
}
}
}
AnimalListViewItem 클래스를 만들어 줘야 합니다. 아래와 같이 Form1 클래스 아래에 ListViewItem을 상속 받은 AnimalListViewItem 클래스를 만들어 줍니다.
// Form1.cs
namespace class_basic
{
public partial class Form1 : Form
{
}
public class AnimalListViewItem : ListViewItem
{
private CAnimal animal;
public CAnimal Animal
{
get { return animal; } set { animal = value; }
}
public AnimalListViewItem(CAnimal anim)
{
this.animal = anim;
}
public override string ToString()
{
return animal.Info();
}
}
}
프로젝트를 우클릭 하여 CAnimal.cs 파일을 하나 만들어 줍니다. 그리고 그 안에 동물과 사람, 강아지와 새에 대한 클래스를 아래처럼 정의해줍니다.
// CAnimal.cs
namespace animals
{
public class CAnimal
{
protected string strName;
public string Name
{
get { return strName; }
set { strName = value; }
}
public CAnimal()
{
strName = "동물";
}
public virtual string Sound()
{
return "소리";
}
public virtual string Move()
{
return "이동";
}
public virtual string Eat()
{
return "먹기";
}
public string Info()
{
return strName;
}
}
public class CPerson : CAnimal
{
public CPerson()
{
strName = "사람";
}
public override string Sound()
{
return "안녕하세요";
}
public override string Move()
{
return "두발로 이동";
}
public override string Eat()
{
return "고기를 구워서 먹기";
}
}
public class CDog : CAnimal
{
public CDog()
{
strName = "강아지";
}
public override string Sound()
{
return "멍멍";
}
public override string Move()
{
return "네발로 이동";
}
public override string Eat()
{
return "사료 먹기";
}
}
public class CBird : CAnimal
{
public CBird()
{
strName = "새";
}
public override string Sound()
{
return "짹짹";
}
public override string Move()
{
return "날개로 이동";
}
public override string Eat()
{
return "벌레 먹기";
}
}
}
상속 관계는 아래와 같습니다.
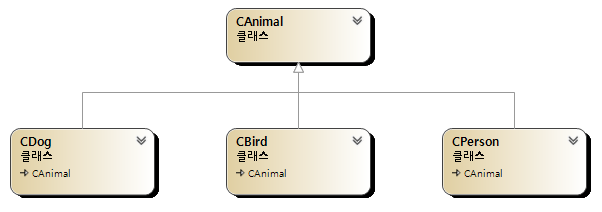
이제 코드를 실행하면 아래와 같이 동작합니다.
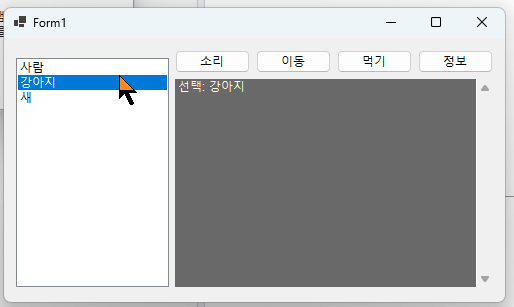
github: https://github.com/3001ssw/c_sharp/tree/main/class_study/class_inheritance